Code Introduction
Line follower
Three infrared sensors are used for the robot and the width of the black line is bigger than the distance between two contiguous sensors. There are thus 4 conditions that can occur and that are represented here below with in red the sensors and in blue the black line :
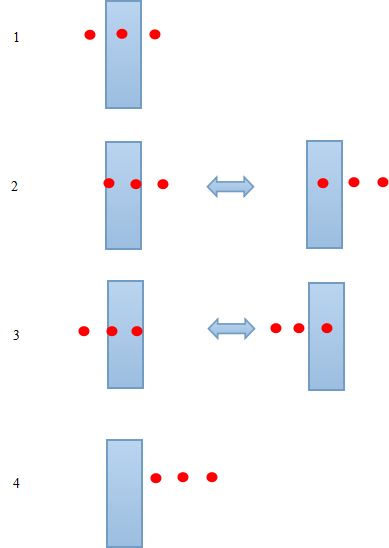
The first condition is the start condition. The robot is positioned on the line and the sensors are calibrated by defining three equal parameters representing the values of the sensors. As already seen before, when a sensor is on a black line, its value will increase, and when it is on a white line it will decrease.
In the second condition, the robot is not axed on the line and should thus turn left. To detect it the Arduino knows the value of the left sensor is bigger than the others and action thus the motors to turn left (done in an if loop).
In the third condition, the robot should turn right since the value of the right sensor is higher.
Finally, in the last condition, all the sensors are on white and thus this mean that there is no black wire to follow and the robot should thus stop. The condition that is coded here is that the value of the middle sensor is smaller than the other one.
void calibrate() {
for (int x=0; x<10; x++) { // run this 10 times to obtain average
digitalWrite(lights, HIGH); // lights on
delay(100);
LDR1 = analogRead(0); // read the 3 sensors
LDR2 = analogRead(1);
LDR3 = analogRead(2);
leftOffset = leftOffset + LDR1; // add value of left sensor to total
centre = centre + LDR2; // add value of centre sensor to total
rightOffset = rightOffset + LDR3; // add value of right sensor to total
delay(100);
digitalWrite(lights, LOW); // lights off
delay(100);
}
// obtain average for each sensor
leftOffset = leftOffset / 10;
rightOffset = rightOffset / 10;
centre = centre /10;
// calculate offsets for left and right sensors
leftOffset = centre - leftOffset;
rightOffset = centre - rightOffset;
}
void goStraight(){
servo_left.write(zero_left-speed1);
servo_right.write(zero_right+speed1);
delay(15); // wait for the servo's to reach it (acceleration time)
}
void goLeft(){
servo_left.write(zero_left-rotate);
servo_right.write(zero_right+speed1+rotate);
delay(15);
}
void goRight(){
servo_left.write(zero_left-speed1-rotate);
servo_right.write(zero_right+rotate);
delay(15);
}
void servos_stop(){
servo_left.write(zero_left);
servo_right.write(zero_right);
delay(15);
}
void setup()
{
Serial.begin(9600);
pinMode(lights, OUTPUT); // output to lights
servo_left.attach(servo_l); // attaches the servo_left on pin 1 to the servo object
servo_right.attach(servo_r); // attaches the servo_right on pin 2 to the servo object
servos_stop();
calibrate();
delay(3000);
digitalWrite(lights, HIGH); // lights on
delay(100);
}
void loop()
{
goStraight();
LDR1 = analogRead(0) + leftOffset;
LDR2 = analogRead(1);
LDR3 = analogRead(2) + rightOffset;
// if LDR1 is greater than LDR2 and LDR3 + threshold turn right
if ((LDR1 > (LDR2+threshhold1))&&(LDR1 > (LDR3+threshhold1))){
goRight();
}
// if LDR3 is greater than LDR2 and LDR1 + threshold turn left
else if ((LDR3 > (LDR2+threshhold1))&&(LDR3 > (LDR1+threshhold1))) {
goLeft();
}
else if ((LDR1 > (LDR2+threshhold1))&&(LDR3 > (LDR2+threshhold1))){
servos_stop();
}
delay(100);
}
The threshold is the difference in values required between the center sensor and the left or right sensors before the robot has to make a turn.
Zero_left and zero_right are the values to send to the wheels if one wants them to stop turning (they were found in practice).
Another remark is also that in our case, one of the motors turns in the other direction than the other one and thus to move forward it is commanded with values under its zero.
WIRE FOLLOWER
The code written for a wire follower is similar to the previous one. But here there are only 2 sensors and only the difference between the two values (computed electronically) is taken as input by the Arduino. Is this value is positive (and higher than a certain threshhold), the robot turns in one direction. If it is negative, it turns in another direction. And if it is near to zero then the robot just moves forward.