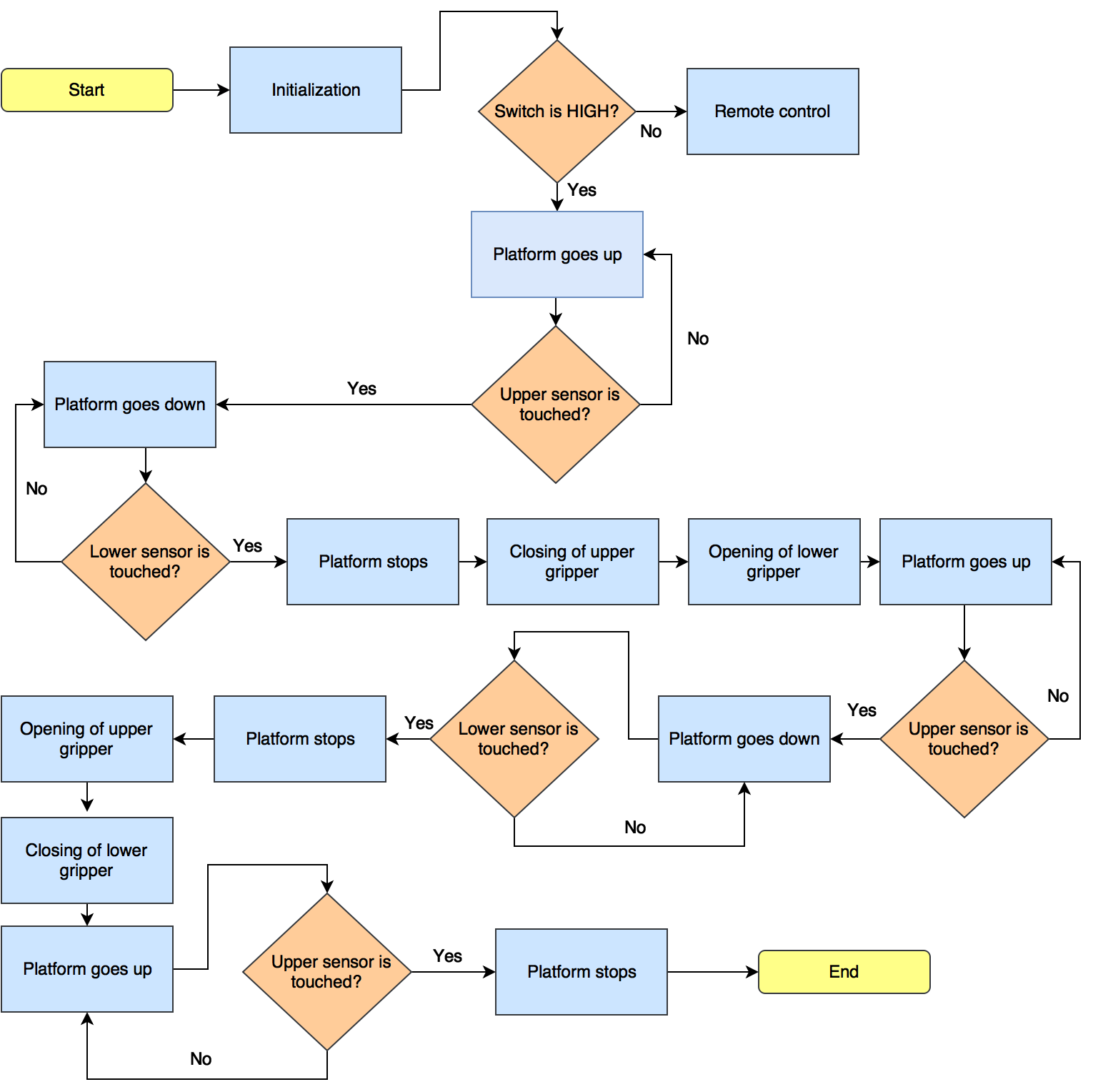
Flow chart
This part of the website is dedicated to the automatic mode, i.e. when the gripper takes the egg and replaces it automatically. The entire code can be read by clicking on the button at the end of the web page.
Once the GrippEgg is started, all the variables are assigned and initialized. Depending on the state of the switch you can either control the grippers and the platform with a remote control or let the gripper take the egg autonomously. As a reminder, the egg is standing in the lower gripper at the beginning.
The platform will by default go upwards until it touches the upper endstop. Once the lower endstop is triggered, the upper gripper attached to the platform will close its fingers while the other gripper will open its. The gripper with the egg will go to the top until the platform touches again the upper endstop.
Finally, the platform will redo the same path to replace the egg back and to return to its rest position.
Arduino code
Variable assignment
First, the digital pins of the Arduino are attributed to the components used such as the stepper, the servo motors, the driver, the endstops and the switch between the automatic mode and the manual one. Then, a value is assigned to a state variable (which corresponds to the first state of the process) because the code is divided in different states.
#include <Servo.h>
//---------------------Servos-------------------------
Servo servoUp;
Servo servoDown;
int positionUp = 190; // Closed angle
int positionDown = 60; //Open angle
//---------------------Stepper------------------------
int Step = 3; //Step pin of the driver
int dir = 2; //Direction pin of the driver
int enable = 4; // Sleep pin of the driver
int ms2 = 6, ms1 = 5; // Microsteps pins of the driver
//---------------------Sensors------------------------
int stateMotion = 1; // 1: Going down and taking the egg, 2: Going up with the egg,
int readEndUp, readEndDown; //3 : Going down and putting the egg back, 4: Going up without the egg
int switchOne = 8; //Switch bewteen manual and automatic
int endStopUp = 13, endStopDown = 12; // Endstops pins
int ManOrAuto; // Manual or automatic mode
int Delay;
Setup
All the variables are initialized and the lower gripper closes its fingers to be sure the egg is laying in it and thus avoiding to break the egg in case of an error in the previous cycle.
void setup()
{
servoUp.attach(11);
servoDown.attach(10);
pinMode(Step, OUTPUT);
pinMode(dir, OUTPUT);
pinMode(enable, OUTPUT);
pinMode(ms1, OUTPUT);
pinMode(ms2, OUTPUT);
digitalWrite(dir, LOW);
pinMode(switchOne, INPUT);
pinMode(readEndUp, INPUT);
pinMode(readEndDown, INPUT);
pinMode(9600);
grip(servoDown, positionUp, 3000); //Close the bottom gripper to be sure the egg is hold
}
Loop
The read variables of the endstops are initialized. The code follows then the states explained in the flow chart.
void loop()
{
readEndUp = digitalRead(endStopUp); // Read the value of the upper endstop
readEndDown = digitalRead(endStopDown); // Read the value of the lower endstop
if (stateMotion == 1) {
movePlatform(); //By default, the platform goes up
if (readEndUp == HIGH))
{
digitalWrite(dir, HIGH); // if the upper endstop is touched the platform goes down
}
if (readEndDown == HIGH)) {
digitalWrite(enable, LOW); // if the bottom endstop is touched the stepper stops
grip(servoUp, positionUp, 10000); // The upper gripper closes its fingers
grip(servoDown, positionDown, 8000); // The bottom gripper opens its fingers
stateMotion = 2;
}
}
if (stateMotion == 2) {
digitalWrite(dir, LOW); //The platforms goes up
movePlatform();
if (readEndUp == HIGH)) { // if the upper endstop is touched
delay(1000);
stateMotion = 3;
}
}
if (stateMotion == 3) {
digitalWrite(dir, HIGH); //The platforms goes down
movePlatform();
if (readEndDown == HIGH)) { // if the bottom endstop is touched
digitalWrite(dir, LOW); // the direction is changed to go to the top
grip(servoDown, positionUp, 13000); //13sec // The bottom gripper closes its fingers
grip(servoUp, positionDown, 10000);// 10sec// The upper gripper opens its fingers
stateMotion = 4;
}
}
if (stateMotion == 4) {
digitalWrite(dir, LOW);
movePlatform();
if (readEndUp == HIGH)) {
stateMotion = 5; // if the upper endstop is touched, the system goes to a rest position
}
}
Move and grip
The two main functions of the code are shown below. Indeed, these functions allow to move the platform by means of the stepper and to close or open the fingers of the grippers using the position of the potentiometer connected to the servo motor.
void movePlatform()
{
digitalWrite(enable, HIGH);
digitalWrite(Step, HIGH);
delay(10);
digitalWrite(Step, LOW);
delay(10);
}
void grip(Servo servo, int angle, int Delay )
{
servo.write(angle);// Send a angle to the servo
delay(Delay); // Add a delay to let the servo reach the position
}